Unit testing with Quarkus and Flyway
Unit testing in Quarkus differs from Spring, particularly due to the absence of annotations like @DirtiesContext
or @Sql
. This means that the entire test suite runs within a single application context, making it challenging to keep tests independent. To address this, you can utilize Flyway, a database migration tool, to manage your test database state effectively.
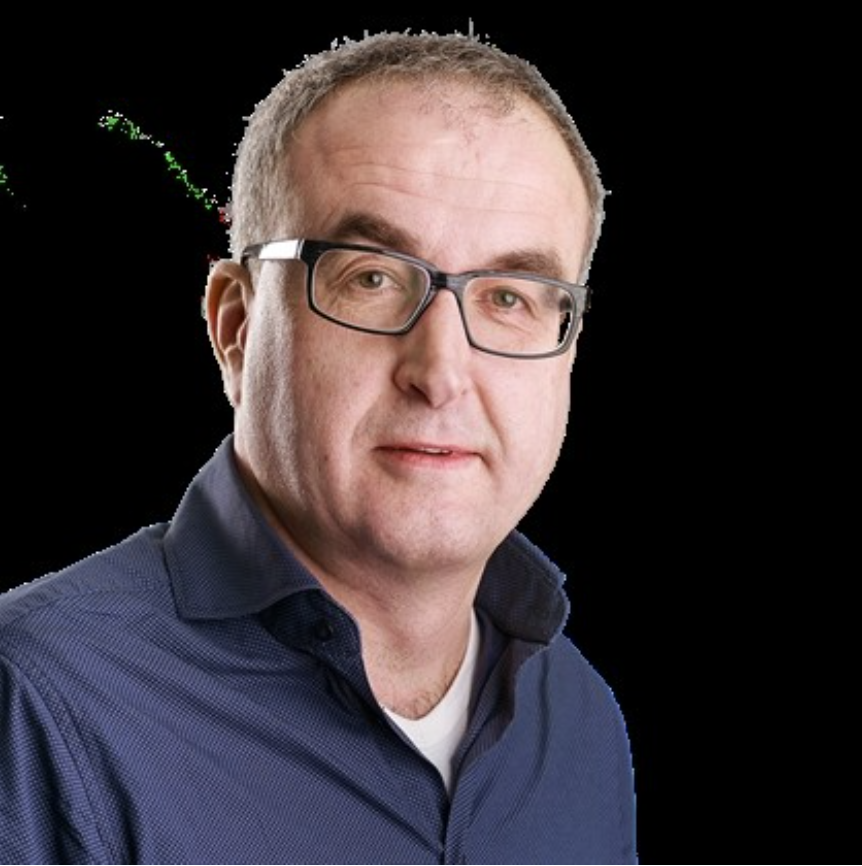
Using Flyway for Test Database Management
Flyway is an open-source tool that handles database migrations and can be leveraged to set up and clean your test database between tests. Here’s how to integrate Flyway into your Quarkus tests:
1. Add Flyway Extension
Include the Flyway extension in your pom.xml:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-flyway</artifactId>
<scope>test</scope>
</dependency>
2 Configure Test Database
In your application.properties for tests, specify the test database configuration:
%test.quarkus.datasource.db-kind=h2
%test.quarkus.datasource.jdbc.url=jdbc:h2:mem:testdb
%test.quarkus.datasource.username=sa
%test.quarkus.datasource.password=
%test.quarkus.flyway.migrate-at-start=true
The %test
prefix ensures that these settings apply only during test execution. The migrate-at-start=true
property instructs Quarkus to run Flyway migrations at the start of each test.
3 Organize Migration Scripts
Place your SQL migration scripts in the src/test/resources/db/migration
directory following Flyway’s naming conventions, such as V1__initial_schema.sql
. These scripts will be executed to set up the database schema before tests run.
4 Writing Tests
Annotate your test classes with @QuarkusTest
to enable Quarkus testing capabilities:
import io.quarkus.test.junit.QuarkusTest;
import org.junit.jupiter.api.Test;
@QuarkusTest
public class FooRepositoryTest {
@Test
public void testFooRepository() {
// Your test code here
}
}
By configuring Flyway to run migrations at the start of each test, you ensure that the database is in a clean state, similar to Spring’s @DirtiesContext
with classMode = BEFORE_EACH_TEST_METHOD
. This approach maintains test isolation and consistency.
Alternative Approach: Testcontainers
Alternatively, you can use Testcontainers to manage your test database lifecycle. Testcontainers allows you to run database instances in Docker containers, providing a fresh database for each test or test class. This ensures complete isolation and eliminates side effects between tests.
By integrating Flyway or Testcontainers into your Quarkus testing strategy, you can maintain independent and reliable unit tests, ensuring a consistent testing environment.