Making Liferay objects work for you: Lessons from real projects
Working with Liferay Objects can be both exciting and frustrating. While they offer a lot of flexibility, we’ve also run into quirks, unexpected behavior, and optimization challenges along the way. After using Objects in real-world projects, we’ve gathered some practical tips—things that surprised us, things we wish we had known earlier, and ways to make Objects work more efficiently. In this post, we’ve compiled practical insights into optimizing Liferay Objects, troubleshooting common issues, and making the most of their capabilities.
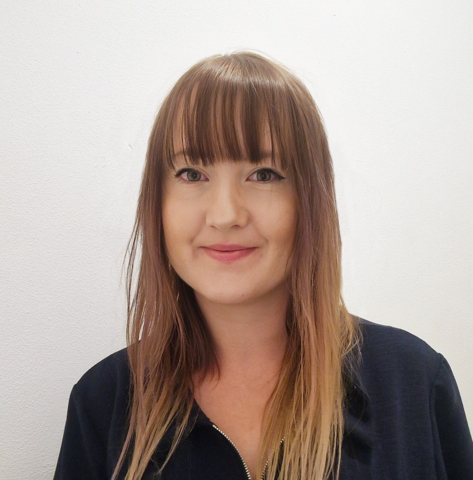
Object Definitions & importing
One of the key aspects of working with Liferay Objects is managing their lifecycle correctly. If you frequently import and update objects, be mindful of the following:
- When using text fields, be sure to choose the correct input length you think is necessary. There are different types of input lengths allowed for certain field types.
- Code-based imports are your friend. Instead of updating objects manually through the interface , look into code strategies to streamline the process and maintain data consistency. For example, using client-extensions like a site-initializer or batch imports. Re-importing Objects can create duplicate entries in the Control Panel.
- Import per Object Definition, not per full directory. This results in clearer error messages and avoids cascading failures across multiple objects.
- Objects may become inactive after reimport. After a reimport, Liferay can set the status to inactive. This means that it also won't be shown as an entry in the panel where you configured it to show. Do not fret, just open the object definition and reset the active status and save.
- External Reference Codes matter. When exporting and importing objects, ensure that external reference codes remain consistent across environments. This prevents mismatched records and ensures better traceability.
Displaying objects in forms
Liferay objects can be shown entered as forms by end-users in different ways. For example, you can use the admin interface, form container fragments or even connect it to Liferay forms. There are some key differences to keep in mind:
- Objects do not support autosave when connected to forms. If you configure a liferay form to use objects as storage, auto-save is not possible.
- Selecting the right storage adapter is crucial. When creating a form, make sure to choose the correct storage adapter for the associated object.
- Mapping required object fields is mandatory. If a form is linked to an object, all required fields must be explicitly mapped, or the submission will fail.
We reccomend using form containers & fragments. A Form container provides more flexibility for complex implementations but requires a bit more manual setup. However, this the recommended futureproof way Liferay recommends. The form container is being improved daily and in Liferay 2025 Q1 contains almost the same functionality as normal forms. Also, it is possible to create your own custom fragments to completely alter the way input is added, which gives you full control on styling, but also autofill possibilities.
Working with Objects via the Headless API
One of the great things of Liferay objects is that there is an automatic headles API for each object. If you need to retrieve Object data via Liferay’s Headless API, be mindful of the following:
- Setting API parameters correctly is essential. Objects expose REST APIs, but fetching the right data efficiently requires proper filtering and pagination. Also, use filtering of fields to minimize data and only retrieve what you use.
- Avoid excessive nested fields. The API allows nested queries to include in the response for objects that are related, but too many nested fields can significantly impact performance. It's better to not use nested queries, unless absolutely necessary.
Using Objects in Java code
For developers integrating Liferay Objects into custom Java code (or groovy scripts), here are some important considerations:
- Understanding site-scoped vs. company-scoped objects is crucial.
- Site-scoped objects use a
groupId
associated with a specific site. - Company-scoped objects use
groupId = 0
, making them globally accessible within the instance.
- Site-scoped objects use a
To fetch object entries in Java from a specific object definition, use Liferay’s built-in services:
@Reference
private ObjectDefinitionLocalService objectDefinitionLocalService;
@Reference
private ObjectEntryLocalService objectEntryLocalService;
ObjectDefinition objectDefinition = objectDefinitionLocalService.fetchObjectDefinition(companyId, externalReferenceCodeOfObjectDefinition);
List<ObjectEntry> objectEntries = objectEntryLocalService.getObjectEntries(groupId, objectDefinition.getObjectDefinitionId(), QueryUtil.ALL_POS, QueryUtil.ALL_POS);
Retrieving the asset entry of this ObjectEntry can be done like this:
AssetEntry assetEntry = assetEntryLocalService.fetchEntry(objectEntry.getModelClassName(), objectEntry.getObjectEntryId());
Or if you want to retrieve Picklists:
@Reference
ListTypeDefinitionLocalService listTypeDefinitionLocalService;
@Reference
ListTypeEntryLocalService listTypeEntryLocalService;
ListTypeDefinition listTypeDefinition = listTypeDefinitionLocalService.fetchListTypeDefinitionByExternalReferenceCode(externalReferenceCodeOfPickListDefinition, companyId);
List<ListTypeEntry> listTypeEntries = listTypeEntryLocalService.getListTypeEntries(listTypeDefinition.getListTypeDefinitionId(), QueryUtil.ALL_POS, QueryUtil.ALL_POS);
And if you want to retrieve a specific list type entry you can get it through it's key:
ListTypeEntry listTypeEntry = listTypeEntryLocalService.fetchListTypeEntry(listTypeDefinition.getListTypeDefinitionId(), key)
Conclusion
Liferay Objects are powerful, but they come with their own set of surprises. These are some of the key lessons we’ve learned while working with Liferay Objects. Hopefully, these tips save you time and frustration! Have you encountered any surprising behaviors or useful workarounds? Let us know—we’re always looking to exchange insights!